Example Web Player¶
The HoloSuite Web Player Example project contains an .html page with a minimal Three.js scene playing HoloStream content. Refer to the Three.js (external link) and HoloStream API documentation for details on individual functions and components.
Overview¶
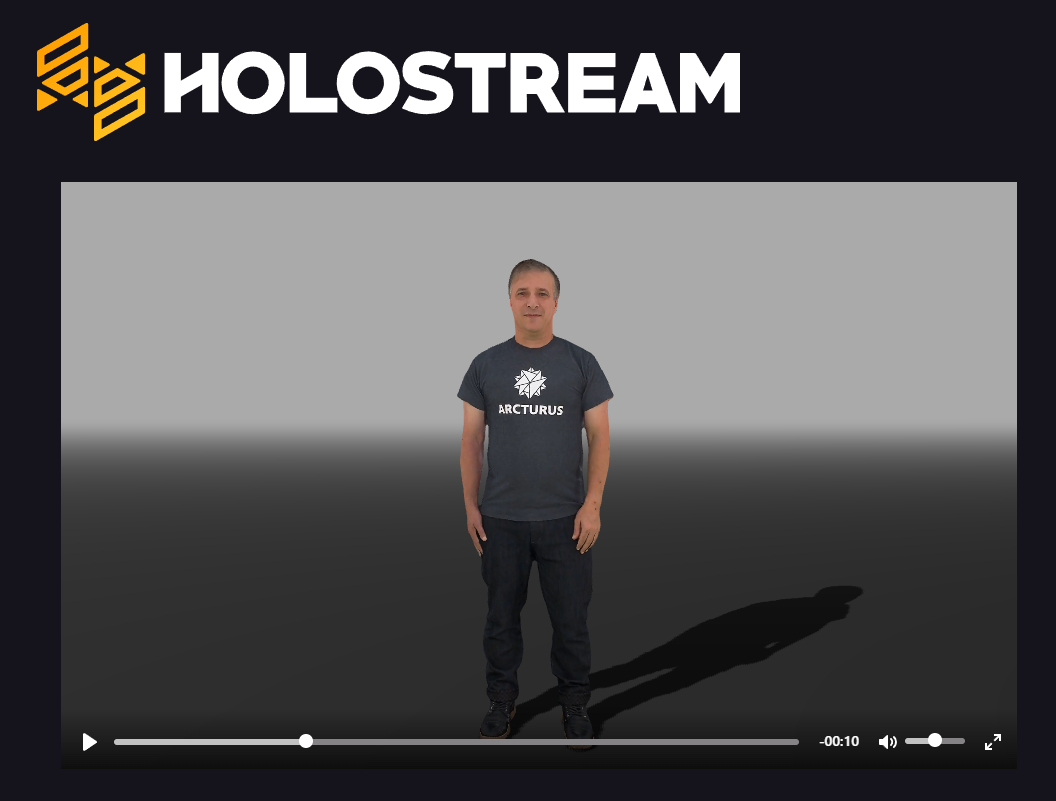
The HoloStream Web Player is configured to stream an example HoloStream clip to a simple scene containing an orbiting camera, depth fog, and shadows, in a player with support for seeking, play/pause, and audio controls.
Location¶
You can find this project under HoloStream-X/HoloStream-WebPlayer-Example-X
Running the project¶
To run the project, simply double click the ExamplePlayer.html file. This will launch the example page in your default browser. The HoloStream player will load and stream an example clip. You can use the playback controls to play, pause and seek through the active content.
Example code explained¶
This section will go over the example code used in ExamplePlayer.html.
Reviewing the project source¶
The Example Web Player is driven by css javascript code in the ExamplePlayer.html file. First, the .css styling specific to the demo page is imported, then the required files for HoloStream are imported:
<link rel="stylesheet" type="text/css" href="https://holostream-static.akamaized.net/release/2021.1.13/remote/holostream-2021.1.13.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r124/three.min.js"></script>
<script src="https://holostream-static.akamaized.net/release/2021.1.13/remote/HoloStream-2021.1.13.min.js"></script>
In the <body>
section, the <script>
tag contains the javascript code driving the example Web Player.
onPageLoad()¶
The example Web Player is initialized in function onPageLoad()
. First, a HoloStream object is constructed. Then, the Three.js scene is configured in initializeScene(holoStream)
. Finally, the holoStream.OpenURL()
function is called to load a HoloStream .mpd URL.
InitializeScene(holoStreamInstance)¶
InitializeScene() is called from onPageLoad(). This function sets up the basic Three.js viewer, containing the HoloStream player, a floor plane, basic lighting, and basic camera controls.
Controlling the scene¶
The holoStream instance exposes a Three.js Scene, Canvas, Renderer, And Camera. These Three.js objects are generated when the holoStream object is created, or existing objects can be supplied to the Constructor. You can edit the Three.js components like you would any other Three.js project.
Fill Color and Fog¶
let fillColor = new THREE.Color("#aaa");
threeObjects.threeScene.background = fillColor;
threeObjects.threeScene.fog = new THREE.FogExp2(fillColor, 0.05);
The scene is configured with a solid background color and exponential fog.
Lighting¶
var light = new THREE.PointLight( 0xffffff, 1, 100 );
light.position.set( -10, 10, 10 );
light.castShadow = true;
light.shadow.mapSize.width = 2048;
light.shadow.mapSize.height = 2048;
threeObjects.threeScene.add( light );
The next section of the example code controls the lighting in the scene. A THREE.PointLight is created with specified color, density, and decay.
Next we set the position of the light, and shadows are enabled and configured. Finally, the light is added to the scene. The HoloStream player used in this example does not receive lighting or shadows, but does cast shadows.
Objects¶
var fillGeometry = new THREE.PlaneGeometry(100, 100, 32);
fillGeometry.translate(0, 0, 0);
fillGeometry.rotateX(-90 / 57.2958);
});
In our sample scene we are only creating one piece of static geometry: the floor plane so that we have something to cast shadows on.
We create this plane by declaring a Three.PlaneGeometry object. The parameters given are width, height, and optionally width segments and height segments. Then the position and rotation (in radians) are set.
var fillMaterial = new THREE.MeshPhongMaterial( {
color: new THREE.Color("#444")
var fillPlane = new THREE.Mesh( fillGeometry, fillMaterial );
fillPlane.receiveShadow = true;
threeObjects.threeScene.add( fillPlane );
Next a phong material is created, configured, and assigned to the plane.
The plane is set to receive shadows, and added to the scene.
Controls¶
let controls = new THREE.OrbitControls( threeObjects.threeCamera, threeObjects.threeRenderer.domElement );
controls.enableDamping = true;
controls.dampingFactor = 0.09;
controls.enableKeys = true;
controls.autoRotate = false;
controls.autoRotateSpeed = 2.0;
controls.target = new THREE.Vector3(0, 1, 0);
controls.maxPolarAngle = Math.PI * .6;
controls.minPolarAngle = Math.PI * .25;
controls.update();
Our example uses Three.js OrbitControls.
Damping is enabled and configured, auto rotate is disabled, and a target and constraints are supplied.