Streaming¶
HoloSuite Player Unity Streaming Overview¶
The Unity HoloStream Player and it’s components are included in the Unity HoloSuite player package. This package contains the scripts and supporting assets necessary to play embedded or streaming OMS clips in your Unity project.
The HoloStream Unity Player is a C# script to download and stream adaptive HoloStream clips directly into a Unity Scene. With further customization users can integrate the HoloStream Player with their Unity scene logic, other scripts and configure dynamic content.
HoloStream Player Install¶
The HoloStream Unity Player is included with the HoloSuite Player Unity Plugin Package, included in your HoloSuite install.
The HoloSuite Player Package supports Unity version 2019.4(LTS). To create a new project using the HoloSuite Player Unity package, first create a new unity project. If you will be working with Unity’s Universal Render Pipeline or High Definition Render Pipeline, create a new project using the appropriate template.
After creating your new project, open the Package Manager window. Click the + button and select Add package from tarball. Navigate to the HoloSuite/Tools folder and select the HoloSuite Player Unity Plugin.tgz
file, then import the package.
The HoloStream Player script¶
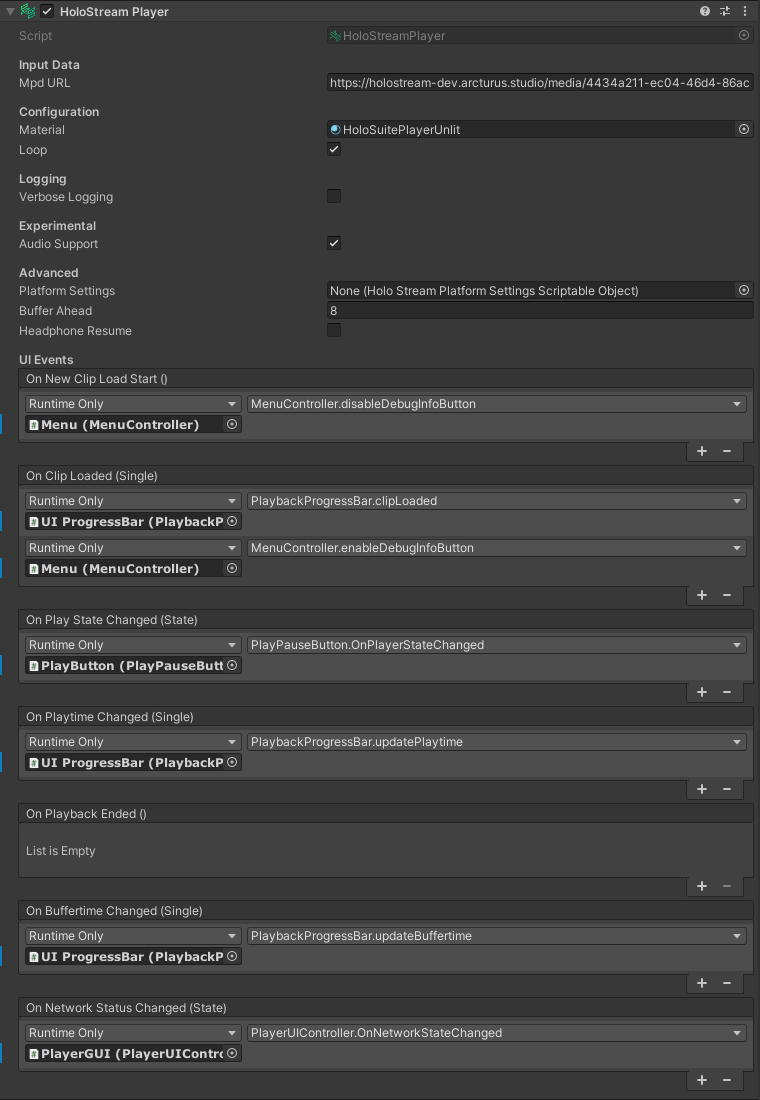
The HoloStream Player script can be applied to any GameObject and configured to turn that object into a HoloStream player.
The HoloStream Player contains the following inspector settings:
Input Data¶
Mpd URL: Supply the .mpd URL for your streaming clip to this parameter. This clip will be loaded and begin streaming when your unity scene is played.
Configuration¶
Material: Set the Material for use in the HoloSuite Player. For proper playback, select a HoloSuite player material appropriate to your render pipeline:
Built-in Render Pipeline: Use the HoloSuite Lit Material or HoloSuite Unlit Material for your Streaming HoloSuite player.
URP: Use the HoloSuite Lit URP Material or the HoloSuite Unlit URP Material for your Streaming HoloSuite player.
HDRP: Use the HoloSuite Lit HDRP Material or HoloSuite Unlit HDRP Material materials for your Streaming HoloSuite player.
Loop: Enable looping playback. If disabled, the clip will freeze after completing playback.
Logging¶
Verbose Logging: Enable console logging for streaming video playback.
Experimental¶
Audio Support: Play any embedded audio in the streaming clip.
Advanced¶
Platform Settings: Supply a Platform Settings scriptable object to specify platform settings.
Buffer Ahead: Set the number of seconds of HoloStream data that can be downloaded ahead of the current time. Must be a positive number.
Headphones Resume: Allows playback to resume automatically when headphones are connected to or disconnected from an iOS device.
HoloStream Platform Settings¶
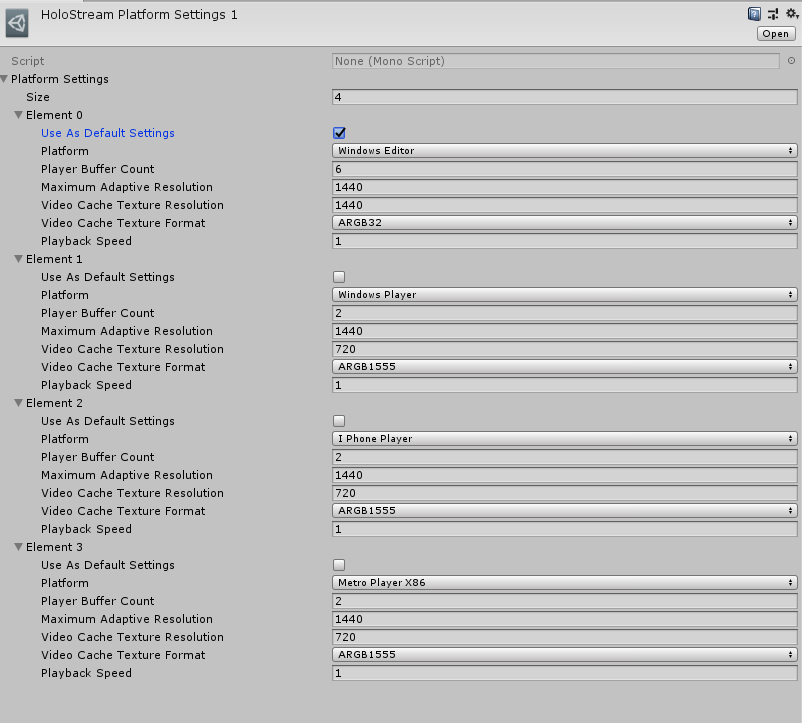
The HoloStream Platform Settings scriptable object is used to override default quality settings for streaming playback. You can create a HoloStream Platform Settings object by right clicking in the Project view and choosing Create > Arcturus > HoloStream > Platform Settings.
The Platform Settings parameter is an array of individual settings. Increase the size to expose one or more platform setting’s elements. Each element has the following parameters:
Use As Default Settings: When there is no setting with a Platform value that matches the current device’s platform, HoloStream will fall back on the Default Settings values. Only mark a single entry as the default settings.
Platform: Choose a supported Unity platform for these platform settings. These settings will be loaded when your HoloStream Unity project is played from a matching device. We recommend only one entry per platform.
Player Buffer Count: The HoloStream player can decode multiple players simultaneously, allowing for smoother playback. The Player Buffer Count sets the number of players that are used for decoding. Decreasing this value could cause playback hitching between SSDR segments, but does reduce load on the device. Inversely, increasing it can smooth hitches, but will increase resource usage for playback. It is not recommended to change this setting from the default value.
Maximum Adaptive Resolution: Set the maximum texture resolution threshold for streaming. Higher resolution profiles will not be requested from the streaming server, even when there is sufficient bandwidth.
Video Cache Texture Resolution: Set the texture resolution used when video frames are cached after download from the streaming server. Larger values may use more memory when playing a streaming clip.
Video Cache Texture Format: Set the Unity texture format used for cached frames.
Playback Speed: Set the playback speed for streaming video. Note that poses will not be interpolated between mesh frames.
The first settings element matching your current platform will be used during HoloStream playback. If no match is found, the last element with “Use As Default Settings” enabled will be used.
Scripting¶
Use the following functions on your HoloStream Player component to programmatically drive streaming playback.
public void Play()¶
Play the currently supplied streaming clip.
public void Stop()¶
Stop playing the current streaming clip.
public void Seek(float targetTime)¶
Seek the specified targetTime
in seconds.
Custom Events¶
Events¶
The following unity events are exposed to interface with HoloStream Playback
UnityEvent OnNewClipLoadStart¶
Invoked immediately when a new clip is loaded via LoadURI()
ClipLoadedEvent onClipLoaded¶
Invoked in the following Update whenever a new clip is loaded via LoadURI()
.
ClipLoadedEvent is a UnityEvent<float>
. The event returns the total duration of the streaming clip.
PlayStateChangeEvent onPlayStateChange¶
Invoked whenever the HoloStream clip starts or stops playing due to LoadURI()
, Play()
, Stop()
, or when playback is paused for buffering.
PlayStateChangeEvent is a UnityEvent<OMSPlayer.State
>. The event returns either the OMSPlayer.State.Play or OMSPlayer.State.Pause state.
PlaytimeChangeEvent onPlaytimeChanged¶
Invoked whenever the current time in the clip changes. This happens every update during uninterrupted playback, and during seeking.
PlaytimeChangeEvent is a UnityEvent<float>
. The event returns the new current Time.
PlaybackEndedEvent onPlaybackEnded¶
Invoked whenever the end of the clip is reached if looping is disabled.
PlaybackEndedEvent is a UnityEvent
.
BufferTimeChangeEvent onBufferTimeChanged¶
Invoked whenever the buffered time reported from the streaming server changes, or when seeking.
BufferTimeChangeEvent is a UnityEvent<float>
. The event returns the new current Time.
NetworkStatusEvent onNetworkStatusChanged¶
Invoked whenever a connection error is reported, or whenever a clip becomes ready for playback or starts buffering.
NetworkStatusEvent is a UnityEvent<StreamRequestWorker.State>
. StreamRequestWorker.State is an enum with the following possible values: ready
, buffering
, connectionerror
.